Headlines
In this Section we will discover a manupulation of the Face Detection using OpenCV and Python, in both using a saved image and trough a webcom streaming.
Code :
From saved image :
import cv2 # Load the cascade face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml') # Read the input image img = cv2.imread('Einstein Group.jpg') # Convert into grayscale gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # Detect faces faces = face_cascade.detectMultiScale(gray, 1.1, 4) # Draw rectangle around the faces for (x, y, w, h) in faces: cv2.rectangle(img, (x, y), (x+w, y+h), (255, 0, 0), 2) # Display the output cv2.imshow('img', img) cv2.waitKey()
From webcam :
import cv2 # Load the cascade face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml') # To capture video from webcam. cap = cv2.VideoCapture(0) # To use a video file as input # cap = cv2.VideoCapture('filename.mp4') while True: # Read the frame _, img = cap.read() # Convert to grayscale gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # Detect the faces faces = face_cascade.detectMultiScale(gray, 1.1, 4) # Draw the rectangle around each face for (x, y, w, h) in faces: cv2.rectangle(img, (x, y), (x+w, y+h), (255, 0, 0), 2) # Display cv2.imshow('img', img) # Stop if escape key is pressed k = cv2.waitKey(30) & 0xff if k==27: break # Release the VideoCapture object cap.release()
Source
https://github.com/salhina/Face-Detection-using-OpenCV-PythonFinal result
- For a prestored image:
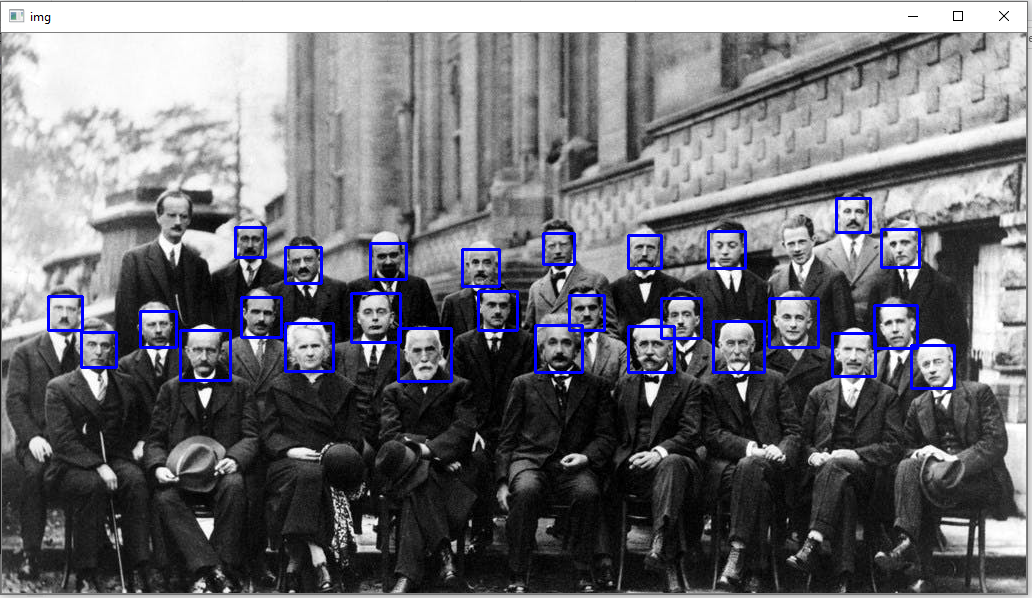