In this manipulation we will see how to Drive a Digital Output hooked into the Arduino via the pin #13, and that by giving it a real-time command from a Tkinter ON/OFF Botton.
Hardware
- Arduino Uno board or any other compatible version.
- LED connected to the pin #13 (or simply the built-in LED).
- Serial medium: USB cable connected to a serial monitor or Pin#0(RX), Pin#1(TX) for Arduino Uno.
Th Code
- Arduino code:
const int ledPin = 13; // the pin that the LED is attached to int incomingByte; // a variable to read incoming serial data into void setup() { // initialize serial communication: Serial.begin(9600); // initialize the LED pin as an output: pinMode(ledPin, OUTPUT); } void loop() { // see if there's incoming serial data: if (Serial.available() > 0) { // read the oldest byte in the serial buffer: incomingByte = Serial.read(); // if it's a capital H (ASCII 72), turn on the LED: if (incomingByte == 'H') { digitalWrite(ledPin, HIGH); } // if it's an L (ASCII 76) turn off the LED: if (incomingByte == 'L') { digitalWrite(ledPin, LOW); } } }
- Python Code
import serial import time import tkinter def quit_button(): global tkTop ser.write(bytes('L', 'UTF-8')) tkTop.destroy() def on_button(): varLabel.set("LED ON ") ser.write(bytes('H', 'UTF-8')) def off_button(): varLabel.set("LED OFF") ser.write(bytes('L', 'UTF-8')) #change the COM port below ser = serial.Serial('COM7', 9600) print("Reset Arduino") time.sleep(2) ser.write(bytes('L', 'UTF-8')) tkTop = tkinter.Tk() tkTop.geometry('300x300') #300 x 300 window tkTop.title("LED control") #name in title bar #label to display the status varLabel = tkinter.IntVar() tkLabel = tkinter.Label(textvariable=varLabel, ) varLabel.set("LED STATUS") tkLabel.pack() #button1 - ON button1 = tkinter.IntVar() button1state = tkinter.Button(tkTop, text="ON", command=on_button, height = 4, width = 8, ) button1state.pack(side='top', ipadx=10, padx=10, pady=15) #button2 - OFF button2 = tkinter.IntVar() button2state = tkinter.Button(tkTop, text="OFF", command=off_button, height = 4, width = 8, ) button2state.pack(side='top', ipadx=10, padx=10, pady=15) #Quit button tkButtonQuit = tkinter.Button( tkTop, text="Quit", command=quit_button, height = 4, width = 8, ) tkButtonQuit.pack(side='top', ipadx=10, padx=10, pady=15) tkinter.mainloop()
The outcoming result
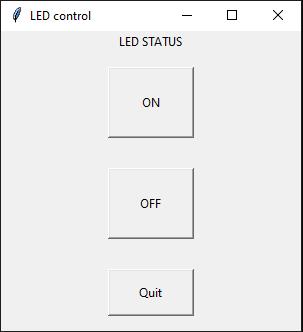